Solving the Error
Encountering errors while programming can be frustrating, especially when dealing with vague or unexpected issues like the “error call to a member function getcollectionparentid() on null”. This error message commonly occurs in object-oriented programming languages like PHP, C#, or Java, where the problem lies in trying to invoke a function on an object that hasn’t been properly initialized. This article will dive into what this error means, the common causes, and how to resolve it with a structured approach.
What Does This Error Mean?
The “error call to a member function getcollectionparentid() on null” indicates that your program is attempting to call the getcollectionparentid()
method on an object that is currently set to null
. In other words, the code is trying to use a function on something that does not exist at the moment.
Breaking it down:
- Call to a Member Function: The program attempts to access a function, in this case,
getcollectionparentid()
, which is a method tied to an object. - On Null: This suggests that the object holding the method is
null
—it either hasn’t been initialized or something went wrong, resulting in no value.
Why Is This Error Occurring?
Understanding why the “error call to a member function getcollectionparentid() on null” happens can help narrow down the troubleshooting process. Below are some common causes:
- Uninitialized Variables: The most typical reason is an object that has not been properly instantiated. Without initialization, the object holds a null reference.
- Null Return Values: Sometimes, functions can return a
null
value when they don’t find what they’re supposed to. When another method is called on this null value without checking for it, this error can occur. - Incorrect Object References: There are cases where the object reference was correct initially, but something changed, making it invalid or null later in the code.
How to Fix the Error
To resolve this error, you need a step-by-step approach that focuses on identifying the null object and addressing the cause. Here are some debugging techniques to help you:
Also Read: Apple Sign Applemiller9to5mac
1. Check for Null References
The first step is to confirm if the object is null at the point where getcollectionparentid()
is being called. You can use debugging tools to print out the object’s value or use conditions to verify it:
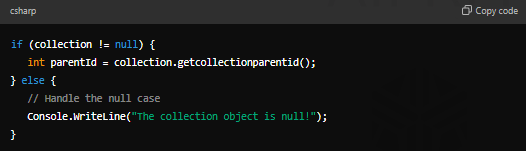
csharpCopy codeif (collection != null) {
int parentId = collection.getcollectionparentid();
} else {
// Handle the null case
Console.WriteLine("The collection object is null!");
}
In the above code, if the collection
is null, it will print a message instead of trying to call the method.
2. Inspect Variable Initialization
Make sure that all necessary objects are initialized before they are used. For example, if you’re working with a collection, ensure the collection is created properly. Missing initialization is one of the top reasons for null reference errors.
3. Review the Function Logic
Sometimes, the function returning the object might not work as expected and could return null under certain conditions. You should thoroughly review your function logic to ensure it handles all possible scenarios.
For instance, if a collection might be empty or missing in certain contexts, your code should account for that:

phpCopy code$collection = getCollectionData();
if ($collection === null) {
// Log the issue or handle it
echo "Collection data is missing!";
} else {
$parentId = $collection->getcollectionparentid();
}
4. Use Null-Safe Operators
Use Null-Safe Operators In some languages like C#, you can use null-safe operators (?.
) to prevent null reference exceptions. This way, if the object is null, the operation will return null instead of throwing an error:

csharpCopy codeint? parentId = collection?.getcollectionparentid();
This ensures that if collection
is null, the program will not attempt to call the function, thus preventing the error.
Common Programming Mistakes Leading to This Error
While working on complex systems, it’s easy to overlook null reference checks. Here are some common programming pitfalls that can lead to the “error call to a member function getcollectionparentid() on null”:
- Missing Validation Checks: Ensure every object is validated before you invoke methods on it.
- Incorrect Data Handling: If your function depends on data from external sources, always handle the possibility that the data might be absent or incorrect.
- Inconsistent Object Lifecycles: If the object’s lifecycle is not properly managed, it can lead to null references. For example, if an object is destroyed or unset unexpectedly, subsequent function calls will fail.
How Debugging Tools Can Help
Modern integrated development environments (IDEs) come with powerful debugging tools. You can step through your code, inspect variables, and pinpoint exactly where and why an object is null. Tools like breakpoints, watches, and step-over functions allow you to monitor your program’s state at different points during execution. If you encounter the “error call to a member function getcollectionparentid() on null”, use these tools to trace the problem back to its origin.
Example in C#
Let’s see how you might fix this issue in a C# program. Consider the following code:

csharpCopy code// Assuming 'collection' is a variable of type 'CollectionClass'
if (collection != null) {
int parentId = collection.getcollectionparentid();
// Now do something with parentId
} else {
// Log or handle the null case
Console.WriteLine("Collection is null, cannot fetch parent ID.");
}
In this case, we check if collection
is null before attempting to call getcollectionparentid()
.
Conclusion
The “error call to a member function getcollectionparentid() on null” is a common and easily solvable issue in object-oriented programming. By taking time to properly initialize variables, check for null values, and debug carefully, you can avoid this frustrating error in your code. Remember, the key to resolving this problem lies in ensuring that you handle objects correctly throughout your program.
This guide has outlined the causes, solutions, and best practices for tackling this issue. With consistent coding habits and mindful debugging, you can avoid running into null reference errors and keep your code running smoothly.